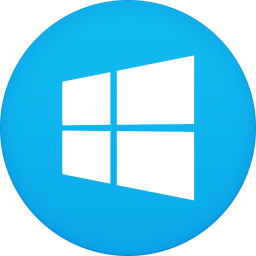
AmCharts
Besides Windows Phone (7.0 and 8.0), the AmCharts API can be used with WPF (Windows Presentation Foundation) and Silverlight applications as well in a similar fashion. It is fast, lightweight and easy-to-use, and supports a variety of charting controls, including Line Charts, Bar Graphs, Area and Pie Charts.
Adding AmCharts to Win Phone app
For this Tutorial, you need Visual Studio 2013 and the Windows Phone 8.0 SDK.
Adding NuGet Packages
- The first step is to install the NuGet Package Manager. Inside Visual Studio, go to Tools >> Extensions and Updates. Now look up NuGet, and you will see the NuGet Package Manager.
You can see that I already have this installed. If you don't, you can install or update it from here. You will need to restart Visual Studio after installing/updating this extension.
- Open Visual Studio again, and then go to Tools >> NuGet Package Manager >> Package Manager Console.
- You will see a console in the output window that is titled 'Package Manager Console', and it will allow you to add some text input. Go ahead and add the following line to it;
Install-Package amChartsQuickCharts
(Note: You need to do the last step while you have an app project open)
The code
Now you have the required packages, and are ready to start coding your charts :).The XAML
Inside the XAML file where you want to add your charts, add this reference in the top Page tag.
xmlns:amCharts="clr-namespace:AmCharts.Windows.QuickCharts;assembly=AmCharts.Windows.QuickCharts.WP"
Inside the XAML, we now need to add the PieChart element.
<amCharts:PieChartx:Name="PC1"TitleMemberPath="title"ValueMemberPath="value" />
This tells the XAML editor to create a <amCharts:PieChart> element, and name it "PC1". For each label-value pair, the name for the label variable would be "label" and the values would be taken from the variable named "val".
That's pretty much it for the visual editor part! Now let's go over to our code-behind file, the file that is named something like 'customName.xaml.cs'.
The C#
Inside the code-behind file, the first thing you want to do is create a custom class for your data. Here's a sample class with the two variables we need defined;
public class PChart {public string label { get; set; }public double val { get; set; }}
Notice that the names of the variables are the same we used in the XAML file. Now we are going to create a collection of PChart objects to bind to our pie chart. You can do this within the main Page class.
public ObservableCollection<PChart> Data = new ObservableCollection<PChart>() {new PChart() { title = "Label 1", value = 30 },new PChart() { title = "Label 2", value = 40 },new PChart() { title = "Label 3", value = 50 },new PChart() { title = "Label 4", value = 60 },
new PChart() { title = "Label 5", value = 70 },
};
You can implement your custom logic here to insert values into the collection. For the purpose of this tutorial, we'll keep it simple. All the above piece of code does is, create new instances of the PChart class, initialize them and then add them to a collection called 'Data'. In the next piece of code, we'll set the source of the pie chart's data to this 'Data'
PC1.DataSource = Data;
Add the above code to the PhoneApplicationPage_Loaded or OnNavigatedTo functions.
You're all done! Run the application, and voila! You'll see a pie chart like so;

The exact same concept applies to Line Charts. You just need to modify the class structure to include more values;
public class LineChart {public string label { get; set; }public double val1 { get; set; }public double val2 { get; set; }public decimal val3 { get; set; }}
And customize the data collection accordingly;
private ObservableCollection<LineChart> _data = new ObservableCollection<LineChart>() {new LineChart() { label = "L1", val1=5, val2=15, val3=12},new LineChart() { label = "L2", val1=15.2, val2=1.5, val3=2.1M},new LineChart() { label = "L3", val1=25, val2=5, val3=2},new LineChart() { label = "L4", val1=8.1, val2=1, val3=22},new LineChart() { label = "L5", val1=8.1, val2=1, val3=22},new LineChart() { label = "L6", val1=8.1, val2=1, val3=22},new LineChart() { label = "L7", val1=4.1, val2=4, val3=2},new LineChart() { label = "L8", val1=8.1, val2=1, val3=22},new LineChart() { label = "L9", val1=8.1, val2=1, val3=22},new LineChart() { label = "L10", val1=8.1, val2=1, val3=22},new LineChart() { label = "L11", val1=8.1, val2=1, val3=22},new LineChart() { label = "L12", val1=8.1, val2=1, val3=22},new LineChart() { label = "L13", val1=4.1, val2=4, val3=2},new LineChart() { label = "L14", val1=8.1, val2=1, val3=22},new LineChart() { label = "L15", val1=8.1, val2=1, val3=22},new LineChart() { label = "L16", val1=8.1, val2=1, val3=22},};
And the XAML;
<amCharts:SerialChart BorderBrush="White" BorderThickness="3" x:Name="chart1" DataSource="{Binding Data}" CategoryValueMemberPath="cat1"
AxisForeground="White"
PlotAreaBackground="Black"
MinimumCategoryGridStep="200"
GridStroke="DarkGray" Margin="0,63,0,10">
<amCharts:SerialChart.Graphs>
<amCharts:LineGraph ValueMemberPath="val1" Title="Line #1" Brush="Blue" />
<amCharts:ColumnGraph ValueMemberPath="val2" Title="Column #2" Brush="#8000FF00" ColumnWidthAllocation="0.4" />
<amCharts:AreaGraph ValueMemberPath="val3" Title="Area #1" Brush="#80FF0000" />
</amCharts:SerialChart.Graphs>
</amCharts:SerialChart>
Note: There are three different types of charts displayed in this XAML; Line, Bar and Area Graphs.
Example;

That's it! You're all done!
You can download the source code for this sample app here. If you have any questions, please feel free to ask in the comments section below - we'd love to help out. Good luck :)
If you don't want to get yourself into Serious Technical Trouble while editing your Blog Template then just sit back and relax and let us do the Job for you at a fairly reasonable cost. Submit your order details by Clicking Here »
Please provide a tutorial about making a simple windows phone app for blogs. Thanks.
ReplyDeleteDear Sir,
ReplyDeleteCould you provide some help with showing X-axis labels.
And is it possible to have bar graph with horizontal bars?